KnockoutJS First Program
KnockoutJS First Program- Let us first create a very basic and simple program in KnockoutJs to understand how it works.
KnockoutJS First Program | Hello World Example
In this tutorial we are going to create a simple example which contains two input fields- Firstname and Lastname. We will print the input box values using KnockoutJs. This example has two parts simply as below –
- 1. View – This contains the html UI parts which will be updated frequently as soon as the data model values are changed.
- 2. View Model – This contains the data model part, this sits behind UI layer and exposes data needed by a View.
View
View Part contains the following code-
KnockoutJs First Example: View
<p>First name: <input data-bind="value: firstName" /></p> <p>Last name: <input data-bind="value: lastName" /></p> <h1>Hello, <span data-bind="text: fullName"> </span>!</h1> |
View Model
View Model Part contains the following code-
KnockoutJs First Example: View Model
<script> // data model content goes here var ViewModel = function() { this.firstName = ko.observable("Lilly"); //It will Assign Default value & keep wathing changes in input field firstName this.lastName = ko.observable("Ray"); //It will Assign Default value & keep wathing changes in input field lastName this.fullName = ko.computed(function() { // Knockout tracks dependencies automatically. It knows that fullName depends on firstName and lastName, because these get called when evaluating fullName. return this.firstName() + " " + this.lastName(); }, this); }; ko.applyBindings(new ViewModel()); </script> |
Complete Example
Now let us combine the both parts and create the full example as below –
| Example:
<!DOCTYPE html> <head> <title>KnockoutJS Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.3.0/knockout-min.js" type="text/javascript"></script> </head> <body> <p>First name: <input data-bind="value: firstName" /></p> <p>Last name: <input data-bind="value: lastName" /></p> <h1>Hello, <span data-bind="text: fullName"> </span>!</h1> <script> // data model content goes here var ViewModel = function() { this.firstName = ko.observable("Lilly"); //It will Assign Default value & keep wathing changes in input field firstName this.lastName = ko.observable("Ray"); //It will Assign Default value & keep wathing changes in input field lastName this.fullName = ko.computed(function() { // Knockout tracks dependencies automatically. It knows that fullName depends on firstName and lastName, because these get called when evaluating fullName. return this.firstName() + " " + this.lastName(); }, this); }; ko.applyBindings(new ViewModel()); </script> </body> </html> |
If you run the above example it will produce output something like this-
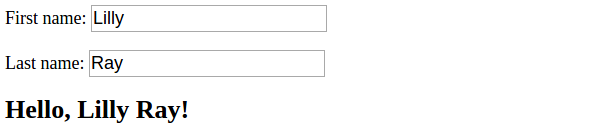
Advertisements
Add Comment
📖 Read More
- 1. KnockoutJS Architecture
- 2. KnockoutJS Observables
- 3. KnockoutJS Observable Array
- 4. KnockoutJS Computed Observables