Category Archives: Vue.Js Blog
Vue.js Array Reverse
This method reverses the order of elements in array. Here in this tutorial, we are going to explain how to use Array reverse in Vuejs. You can also use our online editor to edit and run the code online.
Vue.js Array Reverse Method Example
You can use JavaScript Reverse method simply as below-
Output of above example-

Vue.Js Array Pop
Array Pop function is used to remove the last element from array and it returns that element. Here in this tutorial, we are going to explain how to use native JavaScript Array Pop function remove an element from Array. You can also use our online editor to edit and run the code online.
Vue.Js Array Pop Method Example
You can use JavaScript Array Pop method in Vue.Js simply as below-
Output of above example-
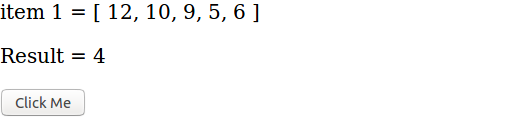
Vue.Js Array Map
This method created a new array by calling a function for each element. Here in this tutorial, we are going to explain how to use JavaScript Array Map function in vue.js. You can use our online editor to edit and run the code online.
Vue.Js Array Map Method Example
You can use Array Map function in vue.js simply as below-
Output of above example-
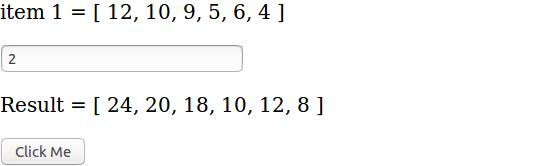
Vue.js Convert Array to String
We can use Native JavaScript join() method to convert an array to string. Here in this article, we are going to explain how you can use this method to join array. You can also use our online editor to edit and run the code online.
Vue.js Convert Array to String- Array Join Method Example
You can convert an array to string simply as below-
Output of above example-

Vue.js check isArray
This method checks whether an object or string is array or not. We can use this method same as native JavaScript. Here in this tutorial, we are going to explain how to check an object is array or not. You can use our online editor to edit and run the code online.
Vue.js check if Object is Array Example
You can check that an object is array or not simply as below-
The above function returns true if an object is array else it will return false.
Output of above example-

Vue.js Array IndexOf
The indexOf method is used to search an specified item in array and returns the position of item. This method returns -1 if item is not found else the position of an item. If occurrence of item is more than once, it will return the index of first index. Here in this tutorial, we are going to explain how to use indexOf Method in vue.js. You can also use our online editor to edit and run the demo online.
Vue.js Array IndexOf Method Example
Here is an example of IndexOf Method in Vue.js-
Output of above example-

Vue.js Array ForEach
We can also use native JavaScript for Each array function in vue.js. Here in this tutorial we are going to explain how to use native for each loop in vuejs. You can also use our online editor to edit and run the code online.
Vue.js Array ForEach Method Example
You can use ForEach loop in vue.js simply as below-
Output of above example-
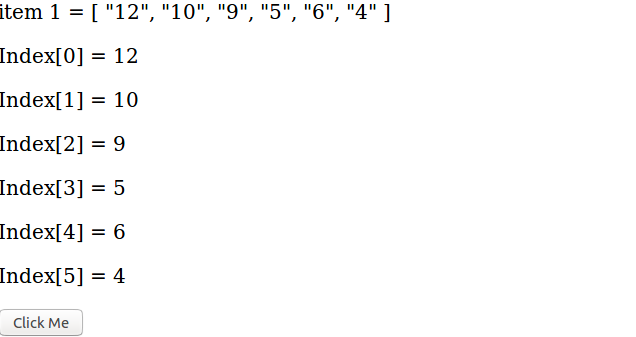
Vue.js Array findIndex
JavaScript function findIndex returns the index of first element which pass the test based on function. Here in this tutorial, we are going to explain how you can use this method to find the index of first element based on the provided function to check the array elements. You can also use our online editor to edit and run the example online.
Vue.js Array findIndex Method Example
You can get index of array element based on test in vuejs simply as below-
Output of above example-

Vue.js Array Find
JavaScript Find method is used to find the value in array based on test(provided as function). Here in this tutorial, we are going to explain how you can use this method in Vuejs. You can also use our online editor to edit and run the code online.
Vue.js Array Find Method Example
You can use find method in vue.js simply as below-
The find function will test the array value based on function checkNum and return the first matching value.
Output of above example-
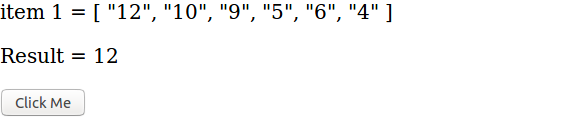