Category Archives: Vue.Js Blog
Vue.js Parse Json
We can use JSON.parse to parse json string in vue.js. Here in this tutorial, we are going to explain how you can use this method to parse json data in vue.js. You can also use our online editor to edit and run the code online.
Vue.js Parse Json JavaScript | VueJS Example
You can parse Json data in vuejs simply as below –
Example:
var jsonData = '{ "id": "0", "name": "Jhon", "email": "jhon@gmail.com" }' // now parse json simply var result = JSON.parse(jsonData); |
Vue.js get dropdown selected value
You can get seelcted text or selected option value of a select box in vue.js using v-model. Here in this tutorial, we are going to explain how you can get selected option value and text in vue.js. You can use our online editor to edit and run the code online.
Vue.js get dropdown selected value JavaScript
Here is an example of simple dropdown options-
Output of above example-
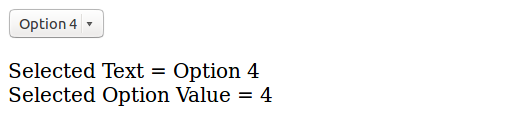
Vue.js check undefined Object or Array
We can use JavaScript typeof operator to check whether an object, array or string is undefined. Here in this tutorial, we are going to explain how you can check undefined objects, array or string in vue.js.
Vue.js check undefined Object or Array JavaScript Example
You can check undefined Object or Array simply as below-
Example:
//check undefined Array if (typeof myArray === "undefined") { alert("myArray is undefined"); } // check undefined object if (typeof myObj === "undefined") { alert("myObj is undefined"); } //check object property if (typeof myObj.some_property === "undefined") { alert("some_property is undefined"); } |
Vue.js decode URL
We can use decodeURIComponent function to decode URL. Here in this tutorial, we are going to explian how you can use this method to decode URL in vue.js. You can also use our online editor to edit and run the code online.
Vue.js decode URL Example
You can use decodeURIComponent to decode the url in vue.js.
Output of above example-

Vue.js encode URL
We can use JavaScript Native JavaScript in-built function encodeURIComponent to encode url in vuejs. Here in this tutorial, we are going to explain how you can use this method to encode url. You can also use our online editor to edit and run the code online.
Vue.js encode URL JavaScript Example
You can use encodeURIComponent function in vue.js simply as below –
Output of above example-

Vue.Js get current timestamp
We can use Date().getTime() method to get the current timestamp in vue.js. Here in this tutorial, we are going to explain how you can get current timestamp in UNIX. You can also use our online editor to edit and run the code online.
Vue.Js get current timestamp Example
You can get current UNIX timestamp in Vue.js simply as below-
Output of above example-

Vue.js check variable is array or object
You can check whether a variable is array or object using native JavaScript typeOf check. Here in this tutorial, we are going to explain how you can use this check in vue.js to decide if variable is array or object.
Vue.js check variable is array or object JavaScript Example
You can check variable is object or array in Vue.js simply as below-
Output of above example-

Vue.js get location pathname
We can use location pathname property to get the current pathname in Vue.js. Here in this tutorial, we are going to explain how you can get location pathname in Vue.js. You can also use our online editor to edit and run the code online.
Vue.js get location pathname JavaScript Example
You can get the location.pathname simply as below-
Output of above example-
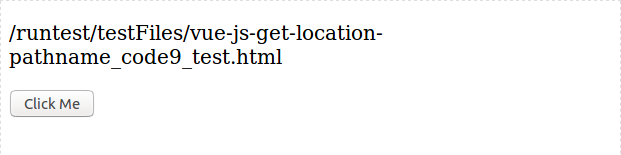
Vue.js get Hostname
Native JavaScript location hostname property to get the hostname. Here in this tutorial, we are going to explain how you can get hostname in vue.js. You can also use our online editor to edit and run the code online.
Vue.js get Hostname Example
You can use location.host to get and set the hostname in vue.js-
Output of above example-
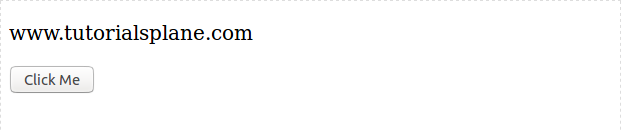